Build apps faster with @occtoo/destination-client
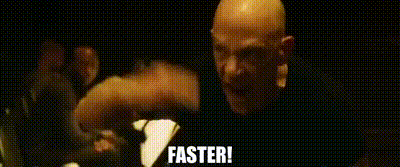
Terrance Fletcher, the ruthless music instructor in the movie Whiplash, once said, "There are no two words in the English language more harmful than 'good job'." While Fletcher's approach may be a bit extreme, the sentiment behind his words is clear: we should always strive to be better, to push ourselves to new heights, and to never settle for mediocrity. This philosophy is especially relevant in the world of software development, where innovation and continuous improvement are essential for success.
At Occtoo, we're committed to helping developers build better apps faster. Our platform provides a powerful set of tools and services that streamline the development process, enabling teams to deliver high-quality applications quickly and efficiently. One of the key components of our platform is the Occtoo Destination service, which allows developers to connect to various data sources and retrieve data from destination endpoints.
To make it even easier for developers to interact with destination
endpoints, we've created the @occtoo/destination-client
npm package. This package simplifies the process of fetching data from destination endpoints, providing an intuitive API and automatic TypeScript typings generation. In this blog post, we'll explore the features of the @occtoo/destination-client
package and show you how to use it to build apps faster and more efficiently.
What is @occtoo/destination-client
?โ
The @occtoo/destination-client
npm package is designed to simplify interactions with your data. Occtoo's platform enables seamless data integration across various systems, and this package acts as a client to efficiently retrieve data from these destinations. It ensures that developers can easily access and work with their data, enhancing productivity and streamlining development workflows.
Core concept - Studio-first, schema-firstโ
Occtoo Studio provides a powerful UI that allows you to create destination endpoints effortlessly, without writing any code. This user-friendly interface lets you define data structures, including fields, types, and relationships with data from various systems.
After defining your destination endpoint in Occtoo Studio, you can use the @occtoo/destination-client package to generate a client. This package automatically creates TypeScript typings based on the schema defined in Occtoo Studio, ensuring type safety and enhancing code quality.
The advantage of this approach is that all type updates and changes can be made through the Occtoo Studio UI. Whenever you modify the schema, you can simply regenerate the client using the @occtoo/destination-client package. This ensures that your client stays up-to-date with the latest schema changes, eliminating the need for manual typings generation or tedious code updates.
Working schema-first with Occtoo Studio and @occtoo/destination-client offers a streamlined and efficient method for integrating with various systems. It removes the need for manual typing and provides a visual interface for managing data structures and mappings. This approach lets you focus on building your application logic, not having to deal with the code complexities of data integration.
Key Featuresโ
- Quick Setup: Set up the client in minutes with minimal configuration ๐ถ๏ธ
- TypeScript Support: Automatically generates necessary TypeScript typings, enhancing code quality and development speed ๐ถ๏ธ
- Easy Regeneration: Easily regenerate the client to adapt to changes in the destination endpoint configurations ๐ถ๏ธ
- Intuitive API: Provides a straightforward API for fetching data ๐ถ๏ธ
- Small package size: The generated client is small in size, providing a minimal footprint in your application source code ๐ถ๏ธ
Installationโ
Prerequisitesโ
Before you start using the @occtoo/destination-client
, you need to have an Occtoo account and a destination endpoint set up in Occtoo Studio. If you don't have an account, you can sign up for a free trial free trial to get started.
In this guide, we'll assume that you already have a React and TypeScript app up and running. The code examples provided below use an empty template created with Next.js, specifically utilizing the latest React Server Components feature. However, please note that the @occtoo/destination-client
can be utilized in almost any JavaScript or TypeScript project.
To begin using @occtoo/destination-client
, install it via npm:
npm install @occtoo/destination-client
Client setupโ
Setting up the @occtoo/destination-client
in your project is incredibly fast and easy. Hereโs how you can get started:
-
๐ Add your destination config
First, put your Occtoo Destination values in your
.env
file. If it doesn't exist, create one in the root of your project. Add the following values:OCCTOO_DESTINATION_ID=...
OCCTOO_DESTINATION_URL=...The destination config can be found in the Occtoo Studio UI. For demo purposes if you don't have a destination, you can use the following values:
OCCTOO_DESTINATION_ID=8903b5d2-9b7f-4297-a04e-a3d339187389
OCCTOO_DESTINATION_URL=https://global.occtoo.com/occtoodemo/occtooFrontEndDocumentation/v3